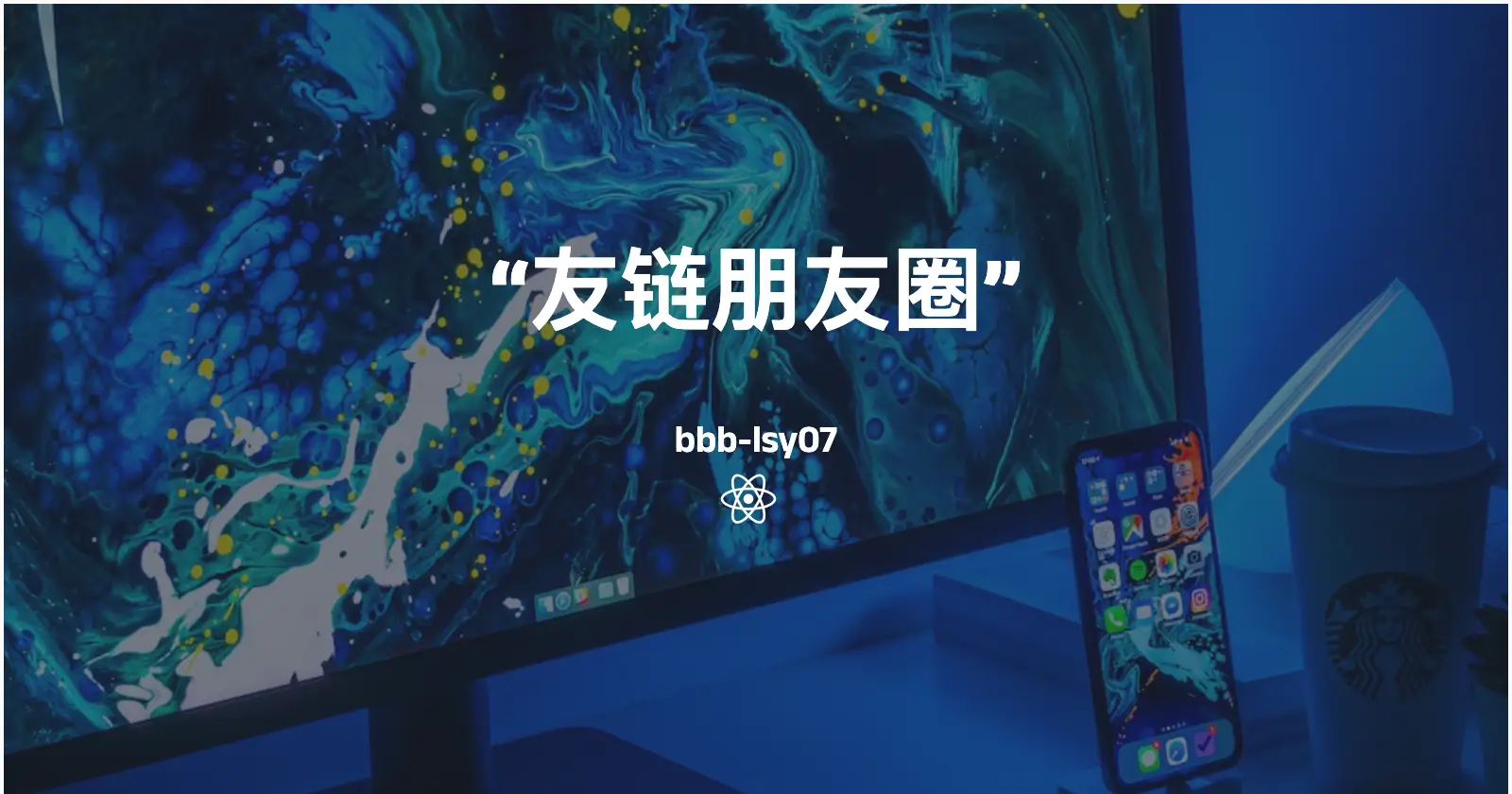
简单版“友链朋友圈”
本文最后更新于 2025-03-02,距离发布已超过7天,文章内容可能已经过时,不保证准确性。
简单版“友链朋友圈”开发
前言:
发现“友链朋友圈”配置都比较复杂,于是决定自己开发一款渐变轻量级的友链朋友圈。
点右侧的文章目录快速跳转到版本
都有详细的注释,你可以进行二次开发,使用代码希望您能注明来自https://i.bbb-lsy07.sbs/,谢谢!
1.0.0
非常轻便,简单上手
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
.friend {
border: 1px solid #ccc;
padding: 10px;
margin-bottom: 20px;
max-width: 600px;
}
.friend img {
width: 50px;
height: 50px;
margin-right: 10px;
vertical-align: middle;
}
.friend h2 {
margin: 0;
display: inline;
font-size: 20px;
}
.friend p {
margin: 5px 0;
color: #666;
}
.friend ul {
list-style-type: none;
padding: 0;
}
.friend li {
margin-bottom: 10px;
}
.friend a {
text-decoration: none;
color: #007bff;
}
.friend a:hover {
text-decoration: underline;
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach(friend => {
// 创建朋友的展示区域
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
<p>${friend.description}</p>
<ul id="articles-${friend.name.replace(/\s+/g, '-') }">
<!-- 文章列表将动态插入这里 -->
</ul>
`;
container.appendChild(friendDiv);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
// 显示最新的 5 篇文章
data.items.slice(0, 5).forEach(item => {
const li = document.createElement('li');
li.innerHTML = `<a href="${item.link}" target="_blank">${item.title}</a>`;
articlesList.appendChild(li);
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
</script>
</body>
</html>
1.0.1
增加动画和一些样式
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 30px;
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: all 0.3s ease;
opacity: 0;
transform: translateY(20px);
}
.friend.loaded {
opacity: 1;
transform: translateY(0);
animation: slideUp 0.5s ease-out;
}
.friend:hover {
transform: translateY(-5px);
border-color: #3498db;
box-shadow: 0 6px 20px rgba(0, 0, 0, 0.15);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
}
.friend h2 a {
color: #3498db;
text-decoration: none;
}
.friend h2 a:hover {
text-decoration: underline;
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
animation: fadeIn 0.3s ease forwards;
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
}
.article-title:hover {
color: #2980b9;
text-decoration: underline;
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: background-color 0.3s;
}
.load-more:hover {
background-color: #2980b9;
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
}
.load-more.loading::after {
content: " ⏳";
animation: spin 1s linear infinite;
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
}
@keyframes slideUp {
from { opacity: 0; transform: translateY(20px); }
to { opacity: 1; transform: translateY(0); }
}
@keyframes fadeIn {
from { opacity: 0; }
to { opacity: 1; }
}
@keyframes spin {
from { transform: rotate(0deg); }
to { transform: rotate(360deg); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach(friend => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;">加载更多</button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画
setTimeout(() => friendDiv.classList.add('loaded'), 100);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.style.animationDelay = `${index * 0.1}s`;
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.textContent = '加载中';
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.textContent = '加载更多';
loadMoreBtn.disabled = false;
}, 800); // 模拟加载延迟
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
// 找到最后一个完整的句子(标点符号)
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
1.0.2
增加更炫酷的动画
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
overflow-x: hidden;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 30px;
animation: fadeInTitle 1.5s cubic-bezier(0.36, 0, 0.66, -0.56) forwards; /* 更柔和的弹簧 */
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
position: relative;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: all 0.6s cubic-bezier(0.5, -0.5, 0.5, 1.5); /* 平滑超弹簧 */
opacity: 0;
transform: translateY(60px) scale(0.92); /* 调整初始位置和缩放 */
position: relative;
overflow: hidden;
}
.friend::before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(45deg, transparent, rgba(52, 152, 219, 0.15), transparent);
opacity: 0;
transform: translateX(-100%);
transition: opacity 0.4s ease-in-out, transform 0.6s ease-in-out;
}
.friend:hover::before {
opacity: 1;
transform: translateX(100%); /* 光晕扫过效果 */
}
.friend.loaded {
opacity: 1;
transform: translateY(0) scale(1);
animation: slideUpScale 1.4s cubic-bezier(0.5, -0.5, 0.5, 1.5); /* 更细腻的弹簧 */
}
.friend:hover {
transform: translateY(-5px) scale(1.03); /* 调整 hover 幅度 */
border-color: #3498db;
box-shadow: 0 8px 20px rgba(0, 0, 0, 0.2);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
position: relative;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
transition: transform 0.5s cubic-bezier(0.36, 1.5, 0.64, 1); /* 更细腻的头像动画 */
}
.friend:hover img {
transform: scale(1.1) rotate(8deg); /* 调整旋转幅度 */
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
position: relative;
}
.friend h2::after {
content: '';
position: absolute;
bottom: -5px;
left: 50%;
width: 0;
height: 2px;
background: #3498db;
transform: translateX(-50%);
transition: width 0.4s cubic-bezier(0.5, 0, 0, 1); /* 从中间展开 */
}
.friend h2:hover::after {
width: 80%; /* 下划线更柔和 */
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
animation: fadeInText 1s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
transform: translateY(20px) scale(0.98); /* 从下方滑入,调整方向 */
animation: fadeInSlide 0.9s cubic-bezier(0.36, 1.5, 0.64, 1) forwards; /* 更细腻弹簧 */
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
position: relative;
transition: all 0.4s ease-in-out;
}
.article-title::before {
content: '✦'; /* 改为小星星装饰 */
margin-right: 6px;
opacity: 0;
transform: scale(0);
transition: all 0.3s cubic-bezier(0.5, 1.5, 0.5, 1);
}
.article-title:hover::before {
opacity: 1;
transform: scale(1); /* 星星弹入 */
}
.article-title:hover {
color: #2980b9;
transform: translateX(5px); /* 轻微右移 */
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
animation: fadeInText 1.2s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
animation: fadeInText 1.4s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: all 0.5s cubic-bezier(0.36, 1.5, 0.64, 1);
position: relative;
overflow: hidden;
}
.load-more::before {
content: '';
position: absolute;
top: 50%;
left: 50%;
width: 0;
height: 0;
background: rgba(255, 255, 255, 0.4);
border-radius: 50%;
transform: translate(-50%, -50%);
transition: width 0.5s ease-out, height 0.5s ease-out;
}
.load-more:hover::before {
width: 150px;
height: 150px; /* 涟漪缩小范围 */
}
.load-more:hover {
background-color: #2980b9;
transform: scale(1.08) translateY(-2px); /* 调整幅度 */
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
transform: scale(1);
}
.load-more.loading::before {
display: none;
}
.load-more.loading span {
display: inline-block;
animation: bounceSpin 1.2s ease-in-out infinite; /* 跳跃旋转 */
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
animation: pulse 2.2s infinite cubic-bezier(0.36, 0, 0.66, -0.56); /* 更细腻脉冲 */
}
@keyframes slideUpScale {
0% { opacity: 0; transform: translateY(60px) scale(0.92); }
40% { opacity: 0.7; transform: translateY(-15px) scale(1.04); } /* 第一次反弹 */
70% { opacity: 1; transform: translateY(5px) scale(0.98); } /* 第二次微调 */
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInSlide {
0% { opacity: 0; transform: translateY(20px) scale(0.98); }
60% { opacity: 0.9; transform: translateY(-5px) scale(1.02); } /* 轻微超调 */
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInTitle {
0% { opacity: 0; transform: translateY(-40px) scale(0.9); }
50% { opacity: 0.8; transform: translateY(10px) scale(1.03); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInText {
0% { opacity: 0; transform: translateY(15px); }
70% { opacity: 0.9; transform: translateY(-2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes bounceSpin {
0% { transform: translateY(0) rotate(0deg); }
25% { transform: translateY(-5px) rotate(90deg); }
50% { transform: translateY(0) rotate(180deg); }
75% { transform: translateY(-3px) rotate(270deg); }
100% { transform: translateY(0) rotate(360deg); }
}
@keyframes pulse {
0% { opacity: 0.2; transform: scale(0.9) translateY(5px); }
50% { opacity: 1; transform: scale(1) translateY(-5px); }
100% { opacity: 0.2; transform: scale(0.9) translateY(5px); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach((friend, index) => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;"><span>加载更多</span></button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画,逐一延迟
setTimeout(() => friendDiv.classList.add('loaded'), 250 + index * 450); // 延迟到 450ms
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-', '')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.style.animationDelay = `${(index + 1) * 0.25}s`; // 延迟到 0.25s
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.querySelector('span').textContent = '加载中';
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.querySelector('span').textContent = '加载更多';
loadMoreBtn.disabled = false;
}, 800); // 模拟加载延迟
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
1.0.3
把点击加载后的延长动画更加柔和,增加关键帧
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
overflow-x: hidden;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 30px;
animation: fadeInTitle 1.5s cubic-bezier(0.36, 0, 0.66, -0.56) forwards;
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
position: relative;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: all 0.6s cubic-bezier(0.5, -0.5, 0.5, 1.5), border-color 0.3s ease-in-out;
opacity: 0;
transform: translateY(60px) scale(0.92);
position: relative;
overflow: hidden;
}
.friend::before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(45deg, transparent, rgba(52, 152, 219, 0.15), transparent);
opacity: 0;
transform: translateX(-100%);
transition: opacity 0.4s ease-in-out, transform 0.6s ease-in-out;
}
.friend:hover::before {
opacity: 1;
transform: translateX(100%);
}
.friend.loaded {
opacity: 1;
transform: translateY(0) scale(1);
animation: slideUpScale 1.4s cubic-bezier(0.5, -0.5, 0.5, 1.5);
}
.friend:hover {
transform: translateY(-5px) scale(1.03);
border-color: #3498db;
box-shadow: 0 8px 20px rgba(0, 0, 0, 0.2);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
position: relative;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
transition: transform 0.5s cubic-bezier(0.36, 1.5, 0.64, 1);
}
.friend:hover img {
transform: scale(1.1) rotate(8deg);
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
position: relative;
}
.friend h2::after {
content: '';
position: absolute;
bottom: -5px;
left: 50%;
width: 0;
height: 2px;
background: #3498db;
transform: translateX(-50%);
transition: width 0.4s cubic-bezier(0.5, 0, 0, 1);
}
.friend h2:hover::after {
width: 80%;
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
animation: fadeInText 1s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
overflow: hidden;
transition: max-height 0.8s cubic-bezier(0.36, 0, 0.66, -0.56); /* 平滑扩展 */
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
transform: translateY(20px) scale(0.98);
animation: fadeInSlide 0.9s cubic-bezier(0.36, 1.5, 0.64, 1) forwards;
}
.articles-list li.new {
opacity: 0;
transform: translateY(30px) scale(0.95);
animation: slideInNew 0.8s cubic-bezier(0.36, 0, 0.66, -0.56) forwards;
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
position: relative;
transition: all 0.4s ease-in-out;
}
.article-title::before {
content: '✦';
margin-right: 6px;
opacity: 0;
transform: scale(0);
transition: all 0.3s cubic-bezier(0.5, 1.5, 0.5, 1);
}
.article-title:hover::before {
opacity: 1;
transform: scale(1);
}
.article-title:hover {
color: #2980b9;
transform: translateX(5px);
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
animation: fadeInText 1.2s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
animation: fadeInText 1.4s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: all 0.5s cubic-bezier(0.36, 1.5, 0.64, 1);
position: relative;
overflow: hidden;
}
.load-more::before {
content: '';
position: absolute;
top: 50%;
left: 50%;
width: 0;
height: 0;
background: rgba(255, 255, 255, 0.4);
border-radius: 50%;
transform: translate(-50%, -50%);
transition: width 0.5s ease-out, height 0.5s ease-out;
}
.load-more:hover::before {
width: 150px;
height: 150px;
}
.load-more:hover {
background-color: #2980b9;
transform: scale(1.08) translateY(-2px);
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
transform: scale(1);
}
.load-more.loading::before {
display: none;
}
.load-more.loading span {
display: inline-block;
animation: bounceSpin 1.2s ease-in-out infinite;
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
animation: pulse 2.2s infinite cubic-bezier(0.36, 0, 0.66, -0.56);
}
@keyframes slideUpScale {
0% { opacity: 0; transform: translateY(60px) scale(0.92); }
40% { opacity: 0.7; transform: translateY(-15px) scale(1.04); }
70% { opacity: 1; transform: translateY(5px) scale(0.98); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInSlide {
0% { opacity: 0; transform: translateY(20px) scale(0.98); }
60% { opacity: 0.9; transform: translateY(-5px) scale(1.02); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes slideInNew {
0% { opacity: 0; transform: translateY(30px) scale(0.95); }
50% { opacity: 0.8; transform: translateY(-5px) scale(1.01); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInTitle {
0% { opacity: 0; transform: translateY(-40px) scale(0.9); }
50% { opacity: 0.8; transform: translateY(10px) scale(1.03); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInText {
0% { opacity: 0; transform: translateY(15px); }
70% { opacity: 0.9; transform: translateY(-2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes bounceSpin {
0% { transform: translateY(0) rotate(0deg); }
25% { transform: translateY(-5px) rotate(90deg); }
50% { transform: translateY(0) rotate(180deg); }
75% { transform: translateY(-3px) rotate(270deg); }
100% { transform: translateY(0) rotate(360deg); }
}
@keyframes pulse {
0% { opacity: 0.2; transform: scale(0.9) translateY(5px); }
50% { opacity: 1; transform: scale(1) translateY(-5px); }
100% { opacity: 0.2; transform: scale(0.9) translateY(5px); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach((friend, index) => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;"><span>加载更多</span></button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画,逐一延迟
setTimeout(() => friendDiv.classList.add('loaded'), 250 + index * 450);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const currentHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${currentHeight}px`; // 设置初始高度
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.className = 'new';
li.style.animationDelay = `${(index + 1) * 0.25}s`;
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
const newHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${newHeight}px`; // 平滑扩展到新高度
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
setTimeout(() => articlesList.style.maxHeight = 'none', 800); // 恢复无限高度
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.querySelector('span').textContent = '加载中';
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.querySelector('span').textContent = '加载更多';
loadMoreBtn.disabled = false;
}, 800);
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
1.0.4
动画更加细腻
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
overflow-x: hidden;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 30px;
animation: fadeInTitle 1.8s cubic-bezier(0.34, 1.56, 0.64, 1) forwards; /* 更柔和 */
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
position: relative;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: all 0.8s cubic-bezier(0.68, -0.55, 0.27, 1.55), border-color 0.4s ease-in-out, box-shadow 0.4s ease-in-out;
opacity: 0;
transform: translateY(80px) scale(0.9) rotate(-1deg); /* 增加细节 */
position: relative;
overflow: hidden;
}
.friend::before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(45deg, transparent, rgba(52, 152, 219, 0.15), transparent);
opacity: 0;
transform: translateX(-100%);
transition: opacity 0.5s ease-in-out, transform 0.7s ease-in-out;
}
.friend:hover::before {
opacity: 1;
transform: translateX(100%);
}
.friend.loaded {
opacity: 1;
transform: translateY(0) scale(1) rotate(0deg);
animation: slideUpScale 1.6s cubic-bezier(0.68, -0.55, 0.27, 1.55); /* 更细腻 */
}
.friend:hover {
transform: translateY(-8px) scale(1.04);
border-color: #3498db;
box-shadow: 0 10px 25px rgba(0, 0, 0, 0.25);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
position: relative;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
transition: transform 0.6s cubic-bezier(0.34, 1.56, 0.64, 1);
}
.friend:hover img {
transform: scale(1.15) rotate(10deg);
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
position: relative;
}
.friend h2::after {
content: '';
position: absolute;
bottom: -5px;
left: 50%;
width: 0;
height: 2px;
background: #3498db;
transform: translateX(-50%);
transition: width 0.5s cubic-bezier(0.5, 0, 0, 1);
}
.friend h2:hover::after {
width: 90%;
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
animation: fadeInText 1.2s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
overflow: hidden;
transition: max-height 1s cubic-bezier(0.34, 1.56, 0.64, 1); /* 更柔和扩展 */
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
transform: translateY(25px) scale(0.97);
animation: fadeInSlide 1s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.articles-list li.new {
opacity: 0;
transform: translateY(40px) scale(0.95) rotate(-1deg); /* 增加细节 */
animation: slideInNew 1s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
position: relative;
transition: all 0.5s cubic-bezier(0.5, 0, 0, 1);
}
.article-title::before {
content: '✦';
margin-right: 6px;
opacity: 0;
transform: scale(0) rotate(-45deg);
transition: all 0.4s cubic-bezier(0.5, 1.5, 0.5, 1);
}
.article-title:hover::before {
opacity: 1;
transform: scale(1) rotate(0deg);
}
.article-title:hover {
color: #2980b9;
transform: translateX(8px);
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
animation: fadeInText 1.4s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
animation: fadeInText 1.6s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: all 0.6s cubic-bezier(0.34, 1.56, 0.64, 1);
position: relative;
overflow: hidden;
}
.load-more::before {
content: '';
position: absolute;
top: 50%;
left: 50%;
width: 0;
height: 0;
background: rgba(255, 255, 255, 0.4);
border-radius: 50%;
transform: translate(-50%, -50%);
transition: width 0.6s ease-out, height 0.6s ease-out;
}
.load-more:hover::before {
width: 180px;
height: 180px;
}
.load-more:hover {
background-color: #2980b9;
transform: scale(1.1) translateY(-3px);
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
transform: scale(1);
}
.load-more.loading::before {
display: none;
}
.load-more.loading span {
display: inline-block;
animation: bounceSpin 1.4s ease-in-out infinite; /* 更细腻 */
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
animation: pulse 2.5s infinite cubic-bezier(0.34, 1.56, 0.64, 1);
}
@keyframes slideUpScale {
0% { opacity: 0; transform: translateY(80px) scale(0.9) rotate(-1deg); }
30% { opacity: 0.6; transform: translateY(-20px) scale(1.05) rotate(0.5deg); }
60% { opacity: 0.9; transform: translateY(10px) scale(0.98) rotate(-0.5deg); }
80% { opacity: 1; transform: translateY(-5px) scale(1.02) rotate(0deg); }
100% { opacity: 1; transform: translateY(0) scale(1) rotate(0deg); }
}
@keyframes fadeInSlide {
0% { opacity: 0; transform: translateY(25px) scale(0.97); }
50% { opacity: 0.8; transform: translateY(-8px) scale(1.03); }
75% { opacity: 1; transform: translateY(3px) scale(0.99); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes slideInNew {
0% { opacity: 0; transform: translateY(40px) scale(0.95) rotate(-1deg); }
40% { opacity: 0.7; transform: translateY(-10px) scale(1.04) rotate(0.5deg); }
70% { opacity: 0.9; transform: translateY(5px) scale(0.98) rotate(-0.3deg); }
100% { opacity: 1; transform: translateY(0) scale(1) rotate(0deg); }
}
@keyframes fadeInTitle {
0% { opacity: 0; transform: translateY(-40px) scale(0.9); }
40% { opacity: 0.7; transform: translateY(15px) scale(1.05); }
70% { opacity: 0.9; transform: translateY(-5px) scale(0.98); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInText {
0% { opacity: 0; transform: translateY(15px); }
60% { opacity: 0.8; transform: translateY(-5px); }
85% { opacity: 1; transform: translateY(2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes bounceSpin {
0% { transform: translateY(0) rotate(0deg); }
20% { transform: translateY(-6px) rotate(72deg); }
40% { transform: translateY(0) rotate(144deg); }
60% { transform: translateY(-4px) rotate(216deg); }
80% { transform: translateY(0) rotate(288deg); }
100% { transform: translateY(0) rotate(360deg); }
}
@keyframes pulse {
0% { opacity: 0.2; transform: scale(0.9) translateY(5px) rotate(-1deg); }
50% { opacity: 1; transform: scale(1.02) translateY(-5px) rotate(1deg); }
100% { opacity: 0.2; transform: scale(0.9) translateY(5px) rotate(-1deg); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach((friend, index) => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;"><span>加载更多</span></button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画,逐一延迟
setTimeout(() => friendDiv.classList.add('loaded'), 300 + index * 500);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const currentHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${currentHeight}px`;
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.className = 'new';
li.style.animationDelay = `${(index + 1) * 0.3}s`; // 增加延迟
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
const newHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${newHeight}px`;
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
setTimeout(() => articlesList.style.maxHeight = 'none', 1000); // 与动画时长同步
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.querySelector('span').textContent = '加载中';
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.querySelector('span').textContent = '加载更多';
loadMoreBtn.disabled = false;
}, 1000); // 延长延迟,与动画同步
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
这是最后一个动画非常炫酷的版本
2.0.0
我们发现过多的动画不是必要的,去除了一些动画,减轻体积,修复已知bug
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
overflow-x: hidden;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 30px;
animation: fadeInTitle 1s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
position: relative;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: transform 0.5s cubic-bezier(0.68, -0.55, 0.27, 1.55), box-shadow 0.5s ease-in-out;
opacity: 0;
transform: translateY(20px) scale(0.98); /* 减少偏移量 */
position: relative;
overflow: hidden;
}
.friend.loaded {
opacity: 1;
transform: translateY(0) scale(1);
animation: slideUpScale 0.8s cubic-bezier(0.68, -0.55, 0.27, 1.55); /* 缩短时长 */
}
.friend:hover {
transform: translateY(-5px) scale(1.02);
box-shadow: 0 10px 25px rgba(0, 0, 0, 0.2);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
transition: transform 0.4s cubic-bezier(0.34, 1.56, 0.64, 1);
}
.friend:hover img {
transform: scale(1.1);
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
}
.friend h2 a {
color: #3498db;
text-decoration: none;
transition: color 0.3s ease-in-out;
}
.friend h2 a:hover {
color: #2980b9;
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
animation: fadeInText 0.8s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
overflow: hidden;
transition: max-height 0.7s cubic-bezier(0.34, 1.56, 0.64, 1); /* 缩短时长 */
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
transform: translateY(10px); /* 减少偏移量 */
animation: fadeInSlide 0.7s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.articles-list li.new {
opacity: 0;
transform: translateY(15px); /* 减少偏移量 */
animation: slideInNew 0.7s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
transition: color 0.3s ease-in-out;
}
.article-title:hover {
color: #2980b9;
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
animation: fadeInText 0.9s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
animation: fadeInText 1s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: all 0.4s cubic-bezier(0.34, 1.56, 0.64, 1);
position: relative;
overflow: hidden;
}
.load-more:hover {
background-color: #2980b9;
transform: scale(1.05);
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
transform: scale(1);
}
.load-more.loading span {
display: none;
}
.load-more.loading::after {
content: '';
position: absolute;
top: 50%;
left: 50%;
width: 18px;
height: 18px;
border: 2px solid #fff;
border-top-color: transparent;
border-radius: 50%;
transform: translate(-50%, -50%);
animation: spinLoader 0.8s linear infinite;
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
animation: pulse 2s infinite cubic-bezier(0.34, 1.56, 0.64, 1);
}
@keyframes slideUpScale {
0% { opacity: 0; transform: translateY(20px) scale(0.98); }
30% { opacity: 0.6; transform: translateY(-10px) scale(1.03); }
60% { opacity: 0.9; transform: translateY(5px) scale(0.99); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInSlide {
0% { opacity: 0; transform: translateY(10px); }
40% { opacity: 0.7; transform: translateY(-5px); }
70% { opacity: 0.95; transform: translateY(2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes slideInNew {
0% { opacity: 0; transform: translateY(15px); }
40% { opacity: 0.7; transform: translateY(-5px); }
70% { opacity: 0.95; transform: translateY(2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes fadeInTitle {
0% { opacity: 0; transform: translateY(-20px) scale(0.95); }
40% { opacity: 0.7; transform: translateY(10px) scale(1.02); }
70% { opacity: 0.95; transform: translateY(-5px); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInText {
0% { opacity: 0; transform: translateY(10px); }
50% { opacity: 0.8; transform: translateY(-3px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes spinLoader {
0% { transform: translate(-50%, -50%) rotate(0deg); }
100% { transform: translate(-50%, -50%) rotate(360deg); }
}
@keyframes pulse {
0% { opacity: 0.3; transform: scale(0.95); }
50% { opacity: 1; transform: scale(1); }
100% { opacity: 0.3; transform: scale(0.95); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach((friend, index) => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;"><span>加载更多</span></button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画,逐一延迟
setTimeout(() => friendDiv.classList.add('loaded'), 200 + index * 300);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const currentHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${currentHeight}px`;
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.className = 'new';
li.style.animationDelay = `${(index + 1) * 0.2}s`;
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
const newHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${newHeight}px`;
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
setTimeout(() => articlesList.style.maxHeight = 'none', 700); // 与动画时长同步
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.disabled = false;
}, 700);
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
2.0.1
在顶部增加推荐文章(就是随机文章),修复已知bug
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>朋友圈</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
margin: 0;
padding: 30px;
overflow-x: hidden;
}
h1 {
text-align: center;
color: #2c3e50;
font-size: 2.5em;
margin-bottom: 15px;
animation: fadeInTitle 1s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
/* 随机文章容器 */
.random-articles {
background: rgba(255, 255, 255, 0.9);
border: 1px solid #e0e7ff;
border-radius: 8px;
padding: 8px 15px;
margin: 0 auto 20px auto;
max-width: 800px;
box-shadow: 0 2px 8px rgba(0, 0, 0, 0.05);
height: 30px;
display: flex;
align-items: center;
overflow: hidden;
position: relative;
}
.random-articles span.label {
font-size: 14px;
color: #3498db;
margin-right: 10px;
font-weight: bold;
flex-shrink: 0;
}
.random-articles .articles-wrapper {
display: flex;
overflow-x: auto;
white-space: nowrap;
flex-grow: 1;
scrollbar-width: none; /* 隐藏滚动条 */
-ms-overflow-style: none;
}
.random-articles .articles-wrapper::-webkit-scrollbar {
display: none; /* Chrome/Safari 隐藏滚动条 */
}
.random-articles a {
color: #2c3e50;
font-size: 14px;
text-decoration: none;
margin-right: 20px;
animation: slideInRandom 0.6s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
transition: color 0.3s ease-in-out;
}
.random-articles a:hover {
color: #2980b9;
}
.random-articles .loading-text {
font-size: 14px;
color: #7f8c8d;
}
.friends-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 25px;
position: relative;
}
.friend {
background-color: #fff;
border: 2px solid #e0e7ff;
border-radius: 12px;
padding: 20px;
width: 320px;
box-shadow: 0 4px 15px rgba(0, 0, 0, 0.1);
transition: transform 0.5s cubic-bezier(0.68, -0.55, 0.27, 1.55), box-shadow 0.5s ease-in-out;
opacity: 0;
transform: translateY(20px) scale(0.98);
position: relative;
overflow: hidden;
}
.friend.loaded {
opacity: 1;
transform: translateY(0) scale(1);
animation: slideUpScale 0.8s cubic-bezier(0.68, -0.55, 0.27, 1.55);
}
.friend:hover {
transform: translateY(-5px) scale(1.02);
box-shadow: 0 10px 25px rgba(0, 0, 0, 0.2);
}
.friend-header {
display: flex;
align-items: center;
margin-bottom: 15px;
}
.friend img {
width: 60px;
height: 60px;
border-radius: 50%;
margin-right: 15px;
border: 2px solid #3498db;
transition: transform 0.4s cubic-bezier(0.34, 1.56, 0.64, 1);
}
.friend:hover img {
transform: scale(1.1);
}
.friend h2 {
margin: 0;
font-size: 20px;
color: #2c3e50;
}
.friend h2 a {
color: #3498db;
text-decoration: none;
transition: color 0.3s ease-in-out;
}
.friend h2 a:hover {
color: #2980b9;
}
.friend p {
margin: 5px 0;
color: #7f8c8d;
font-size: 14px;
animation: fadeInText 0.8s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.articles-list {
list-style-type: none;
padding: 0;
margin: 0;
overflow: hidden;
transition: max-height 0.7s cubic-bezier(0.34, 1.56, 0.64, 1);
}
.articles-list li {
margin-bottom: 20px;
padding-bottom: 15px;
border-bottom: 1px dashed #dfe6e9;
opacity: 0;
transform: translateY(10px);
animation: fadeInSlide 0.7s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.articles-list li.new {
opacity: 0;
transform: translateY(15px);
animation: slideInNew 0.7s cubic-bezier(0.34, 1.56, 0.64, 1) forwards;
}
.article-title {
font-weight: bold;
color: #3498db;
text-decoration: none;
display: block;
font-size: 16px;
margin-bottom: 5px;
transition: color 0.3s ease-in-out;
}
.article-title:hover {
color: #2980b9;
}
.article-time {
font-size: 12px;
color: #95a5a6;
margin-bottom: 5px;
animation: fadeInText 0.9s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.article-summary {
font-size: 14px;
color: #34495e;
line-height: 1.5;
animation: fadeInText 1s cubic-bezier(0.5, 0, 0, 1) forwards;
}
.load-more {
background-color: #3498db;
color: #fff;
border: none;
padding: 8px 15px;
cursor: pointer;
border-radius: 6px;
margin-top: 15px;
transition: all 0.4s cubic-bezier(0.34, 1.56, 0.64, 1);
position: relative;
overflow: hidden;
}
.load-more:hover {
background-color: #2980b9;
transform: scale(1.05);
}
.load-more.loading {
background-color: #95a5a6;
cursor: not-allowed;
transform: scale(1);
}
.load-more.loading span {
display: none;
}
.load-more.loading::after {
content: '';
position: absolute;
top: 50%;
left: 50%;
width: 18px;
height: 18px;
border: 2px solid #fff;
border-top-color: transparent;
border-radius: 50%;
transform: translate(-50%, -50%);
animation: spinLoader 0.8s linear infinite;
}
.loading {
text-align: center;
font-size: 14px;
color: #7f8c8d;
animation: pulse 2s infinite cubic-bezier(0.34, 1.56, 0.64, 1);
}
@keyframes slideUpScale {
0% { opacity: 0; transform: translateY(20px) scale(0.98); }
30% { opacity: 0.6; transform: translateY(-10px) scale(1.03); }
60% { opacity: 0.9; transform: translateY(5px) scale(0.99); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInSlide {
0% { opacity: 0; transform: translateY(10px); }
40% { opacity: 0.7; transform: translateY(-5px); }
70% { opacity: 0.95; transform: translateY(2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes slideInNew {
0% { opacity: 0; transform: translateY(15px); }
40% { opacity: 0.7; transform: translateY(-5px); }
70% { opacity: 0.95; transform: translateY(2px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes slideInRandom {
0% { opacity: 0; transform: translateX(20px); }
60% { opacity: 0.9; transform: translateX(-2px); }
100% { opacity: 1; transform: translateX(0); }
}
@keyframes fadeInTitle {
0% { opacity: 0; transform: translateY(-20px) scale(0.95); }
40% { opacity: 0.7; transform: translateY(10px) scale(1.02); }
70% { opacity: 0.95; transform: translateY(-5px); }
100% { opacity: 1; transform: translateY(0) scale(1); }
}
@keyframes fadeInText {
0% { opacity: 0; transform: translateY(10px); }
50% { opacity: 0.8; transform: translateY(-3px); }
100% { opacity: 1; transform: translateY(0); }
}
@keyframes spinLoader {
0% { transform: translate(-50%, -50%) rotate(0deg); }
100% { transform: translate(-50%, -50%) rotate(360deg); }
}
@keyframes pulse {
0% { opacity: 0.3; transform: scale(0.95); }
50% { opacity: 1; transform: scale(1); }
100% { opacity: 0.3; transform: scale(0.95); }
}
</style>
</head>
<body>
<h1>朋友圈</h1>
<div class="random-articles" id="random-articles">
<span class="label">推荐文章:</span>
<div class="articles-wrapper" id="random-articles-wrapper">
<!-- 随机文章将动态插入这里 -->
</div>
</div>
<div class="friends-container" id="friends-container">
<!-- 朋友圈内容将动态插入这里 -->
</div>
<script>
// 定义朋友圈数据
const friends = [
{
name: '楠笙',
url: 'https://blog.nanshengwx.cn/',
logo: 'https://blog.nanshengwx.cn/upload/logo.png',
description: '记录生活与技术,空谈误国,实干兴邦',
rss: 'https://blog.nanshengwx.cn/rss.xml'
},
{
name: 'Mo 的记事簿',
url: 'https://blog.xiowo.net/',
logo: 'https://blog.xiowo.net/img/avatar.png',
description: '万年鸽王,哈哈 OvO',
rss: 'https://blog.xiowo.net/atom.xml'
}
];
// 获取容器元素
const container = document.getElementById('friends-container');
const randomArticlesWrapper = document.getElementById('random-articles-wrapper');
let allArticles = [];
// 从所有 RSS 中获取文章并显示
const fetchAllArticles = async () => {
randomArticlesWrapper.innerHTML = '<span class="loading-text">正在加载...</span>';
const promises = friends.map(friend =>
fetch(`https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`)
.then(response => response.json())
.then(data => data.status === 'ok' ? data.items : [])
.catch(error => {
console.error(`获取 ${friend.name} 的 RSS 数据时出错:`, error);
return [];
})
);
const results = await Promise.all(promises);
allArticles = results.flat();
if (allArticles.length === 0) {
randomArticlesWrapper.innerHTML = '<span>暂无文章</span>';
} else {
displayRandomArticles();
}
};
// 显示随机文章(水平列表)
const displayRandomArticles = () => {
randomArticlesWrapper.innerHTML = '';
const shuffledArticles = allArticles.sort(() => 0.5 - Math.random()).slice(0, 5); // 随机取 5 篇
shuffledArticles.forEach(article => {
const a = document.createElement('a');
a.href = article.link;
a.target = '_blank';
a.textContent = article.title;
randomArticlesWrapper.appendChild(a);
});
};
// 初始化随机文章
fetchAllArticles();
// 为每个朋友创建展示区域并获取 RSS 数据
friends.forEach((friend, index) => {
const friendDiv = document.createElement('div');
friendDiv.className = 'friend';
friendDiv.innerHTML = `
<div class="friend-header">
<img src="${friend.logo}" alt="${friend.name}">
<h2><a href="${friend.url}" target="_blank">${friend.name}</a></h2>
</div>
<p>${friend.description}</p>
<ul class="articles-list" id="articles-${friend.name.replace(/\s+/g, '-') }">
<li class="loading">加载中...</li>
</ul>
<button class="load-more" id="load-more-${friend.name.replace(/\s+/g, '-') }" style="display: none;"><span>加载更多</span></button>
`;
container.appendChild(friendDiv);
// 添加 loaded 类以触发动画,逐一延迟
setTimeout(() => friendDiv.classList.add('loaded'), 200 + index * 300);
// 构造 RSS 转换 API 的 URL
const rssUrl = `https://api.rss2json.com/v1/api.json?rss_url=${encodeURIComponent(friend.rss)}`;
// 获取并解析 RSS 数据
fetch(rssUrl)
.then(response => response.json())
.then(data => {
if (data.status === 'ok') {
const articlesList = document.getElementById(`articles-${friend.name.replace(/\s+/g, '-')}`);
articlesList.innerHTML = ''; // 清除加载中占位符
const loadMoreBtn = document.getElementById(`load-more-${friend.name.replace(/\s+/g, '-') }`);
const articles = data.items;
let displayed = 0;
const batchSize = 3;
// 显示一批文章
const displayBatch = () => {
const currentHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${currentHeight}px`;
const nextBatch = articles.slice(displayed, displayed + batchSize);
nextBatch.forEach((item, index) => {
const pubDate = new Date(item.pubDate).toLocaleDateString('zh-CN');
const summary = getSummary(item.description);
const li = document.createElement('li');
li.className = 'new';
li.style.animationDelay = `${(index + 1) * 0.2}s`;
li.innerHTML = `
<a href="${item.link}" target="_blank" class="article-title">${item.title}</a>
<div class="article-time">${pubDate}</div>
<div class="article-summary">${summary}</div>
`;
articlesList.appendChild(li);
});
displayed += nextBatch.length;
const newHeight = articlesList.scrollHeight;
articlesList.style.maxHeight = `${newHeight}px`;
if (displayed >= articles.length) {
loadMoreBtn.style.display = 'none';
} else {
loadMoreBtn.style.display = 'block';
}
setTimeout(() => articlesList.style.maxHeight = 'none', 700);
};
// 初始显示
displayBatch();
// 加载更多按钮点击事件
loadMoreBtn.addEventListener('click', () => {
loadMoreBtn.classList.add('loading');
loadMoreBtn.disabled = true;
setTimeout(() => {
displayBatch();
loadMoreBtn.classList.remove('loading');
loadMoreBtn.disabled = false;
}, 700);
});
} else {
console.error('无法获取 RSS 数据:', friend.name);
}
})
.catch(error => {
console.error('获取 RSS 数据时出错:', friend.name, error);
});
});
// 改进摘要提取函数,确保完整句子
function getSummary(description) {
if (!description) return '无摘要';
const parser = new DOMParser();
const doc = parser.parseFromString(description, 'text/html');
const text = doc.body.textContent || '';
if (text.length <= 100) return text;
const truncated = text.substring(0, 100);
const lastPunctuation = Math.max(
truncated.lastIndexOf('。'),
truncated.lastIndexOf('!'),
truncated.lastIndexOf('?')
);
if (lastPunctuation > 0) {
return truncated.substring(0, lastPunctuation + 1);
}
return truncated + '...';
}
</script>
</body>
</html>
总结:
点击下面的链接卡片查看具体效果,目前链接里的版本暂未公开,因为代码比较混乱,我会继续优化和改进代码。
有什么想法和建议,或者是bug反馈,欢迎在评论区指出
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 bbb-lsy07
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果